Hey folks, some guys asked me how to make several stuff by scripting so i´m going to show it you now.
1. Getting started
Before you start, you should prepare on the right way. In our case you should get a nice program to script with. I use Notepad (
http://notepad-plus-plus.org/). Then you can look at your map folder already. It´s located here: MTA San Andreasservermodsdeathmatch
esources<mapname>. Here you´ll find a
*.map file and a
meta.xml file.
2. General
Now that you are prepared, you can start. Open your
meta.xml file. There are following tags shown in it: <meta> & <settings>. If you wanna make a script, the map has to know that it has to load it. So you must add following line into your meta file:
<script src="<scriptname>.lua" type="server" />
.(add the line between the <meta> </meta> tags) You see a part there called
"server". There are "server" and "client" tags. For now let it be like it is now. Now start a
new project in your notepad.
3. Scripting
-Change the water color-
Alright, your meta file should contain the script line i posted above already. Now you should have started a new project in the notepad(if you use it). In this project, insert following lines:
red,green,blue,alpha=0,255,204,255
addEventHandler("onClientResourceStart",getResourceRootElement(),function()
setWaterColor(red,green,blue,alpha)
end)
Ok, what´s that all now?red,green,blue,alpha=0,255,204,255 <<< So that is a RPG code. It tells how much red, blue or green the color contains. In my case this is a turquoise. To make a red out of it, just do 255,0,0,255.
addEventHandler - It´s needed for every event you make in your script.
onClientResourceStart - It tells the script when to start the function. Let it be on "onClientResourceStart" for the water color.
setWaterColor - It just tells the script to change the water color. So actualy the script is finished already. Now save it. Click on save as and select *.lua as the format(
IMPORTANT)! Save it in your map folder. Now you only need to edit the meta.xml file:
<meta>
<script src="water.lua" type="client" />
=> As you can see, i named my script water.lua . This line is on the very top of my script, under the <meta> tag. So now your water color should be changed already. If bugs should appear, open with F8 the console and it´ll tell you which bug it is.
-Teleport-
So now we are going to make a teleport which teleports the player his vehicle. Note: Read the step before so you´ll know what to do now.
Ok so make a new script file. It should contain following code:
function sls(hitElement, matchingDimension)
if getElementType(hitElement)=="player" then
local veh = getPedOccupiedVehicle(hitElement)
if (veh) then -- if player was driving
setElementPosition(veh, 3386.8996582031,-1544.2071533203,40.203617095947) -- we are teleporting his car - player will be moved with it -- remember to fill the zeros with position
else
setElementPosition(hitElement, 3386.8996582031,-1544.2071533203,40.203617095947) -- we are moving player
end
end
end
--To create a marker:
local myMarker = createMarker(3306.7529296875,-1526.4263916016,46.305931091309, "arrow", 2, 0, 0, 255, 255)
addEventHandler("onMarkerHit", myMarker, sls)
Also add a new line to your meta.xml file like following:
<script src="move.lua" type="server" />
The type is
server now.
Remember: How to get positions should you already know.
What does it do?The first part only gets if a player is inside a vehicle, or not. Then it sets his and his cars position. As you can see, i have for both the same
x, y, z coordinates. That´s supposed to be so now. The second part creates a marker.
myMarker = createMarker(x-coord.,y-coord.,z-coord.,"TypeOfTheMarker,Size,Red,Green,Blue,Alpha)
Ok that about the functions.
on both
setElementPosition´s add the coordinates you want to teleport a player to. At createMarker you add the coordinates for the marker to be created.
Example how a teleport looks like:
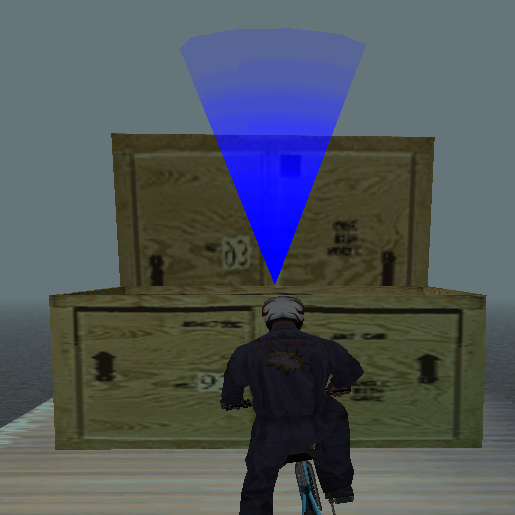
-Moving Objects-
That´s maybe a bit more complicated part of the tutorial. Making moving objects. First you need to make sure that the object isn´t rotated(That makes this process easier now). Then get the x, y,z (and optional rotation) coordinates of the object you want to move. Now make a
new script. First you must tell the script to create a object to move(The one you created before is only there to get the coordinates). This looks like that:
addEventHandler ( "onResourceStart", getRootElement(), move )
function move ()
object1 = createObject ( 10841, 3095.94921875, -1752.4644775391, 34.754978179932)
move1_1 ()
end
createObject(ModelID, x, y, z) <<< Put the rotations behind them if you have some.
move1_1 - That just tells to script to go to the next function which should look like that(put it after the function before):
addEventHandler ( "onResourceStart", getRootElement(), move )
function move1_1 ()
moveObject ( object1, 700, 3095.94921875, -1752.4644775391, 43.754978179932)
setTimer ( move1_2, 700, 1 )
end
function move1_1 - Before, the function went over to this one now. It´s just called move1_1 now.
moveObject(object you created before,time(in milliseconds 1000=1 second),new x, new y, new z) << Again, if you´ve rotations, add them behind it. But it has to be like 0,0,0 , that means x, y,z, and also if you don´t have x,y rotations for example, you´ve to write 0 there, otherwise if you only put the z coordinate there, the script will take it as x and it won´t work well then.
setTimer(new function,Time,1(just write it there, can´t explain it now))
Ok now a other function, which is just a new point of the moving process follows:
addEventHandler ( "onResourceStart", getRootElement(), resource_starts )
function move1_2 ()
moveObject ( object1, 700, 3095.94921875, -1752.4644775391, 34.754978179932)
setTimer ( move1_1, 700, 1 )
end
Actually, it´s the same as above, just that the last line(setTimer) tells the script to go back to the function move1_1 now.
You can put as much moving points as you want, just keep the view over them since it may become confusing then.
-Chat text-
Last chapter of this tutorial: Chat text.
outputChatBox ( "#FF0000---|Burn in #ff9000Hell !|---", getRootElement(), 255, 255, 255, true )
Ok, this is an example from one of my maps. You must put it under a addEventHandler, in my case the event handler is this:
addEventHandler ( "onResourceStart", getRootElement(), resource_starts )
.
So what does it do?It writes a message into the chatbox. Ignore the elements in the back of the line, just focus on this part:
"#FF0000---|Burn in #ff9000Hell !|---"
=> So at the beginning is a hex-color code. You should be able to get them as well. It´s like in the normal chat where you can also put colored messages. So like
#colorcode <text>, that´s all.
AdditionalWater script:
http://www.solidfiles.com/d/76fe0/
Now we are finished. If you have any questions, please ask them here. I hope it helped you

~Tom